猜数字
from random import randint num = randint(1, 100) print('Guess what I think?') bingo = False count = 0 while bingo == False: count += 1 answer = int(input()) if answer<num: print(str(answer)+ ' is too small!') if answer>num: print(str(answer)+ ' is too big!') if answer==num: print('BINGO!%d is the right answer' %answer) bingo = True print(count)
输出-1
* * * * *
* * * * *
* * * * *
* * * * *
* * * * *
for i in range(0, 5): for p in range(0, 5): print('*',end='') print()
输出-2
*
**
***
****
*****
n=0 for i in range(0, 5): for p in range(0, 1+n): print('*',end='') n=n+1 print()
猜数字
def isEqual(num1, num2): if num1<num2: print ('too small') return False if num1>num2: print ('too big') return False if num1==num2: print ('bingo!') return True from random import randint num = randint(1, 100) print('Guess what I think?') bingo = False while bingo == False: answer = int(input()) bingo = isEqual(answer, num)
函数可以把某个功能的代码分离出来,在需要的时候重复使用,就像拼装积木一样,这会让程序结构更清晰。
if的嵌套
if y >= 0: if x >= 0: print (1) else: print (2) else: if x < 0: print (3) else: print (4)
点球小游戏
待会儿要去现场看场中超比赛。于是想到,以我们现在所学的,可以做一个罚点球的游戏。大概流程是这样: 每一轮,你先输入一个方向射门,然后电脑随机判断一个方向扑救。方向不同则算进球得分,方向相同算扑救成功,不得分。 之后攻守轮换,你选择一个方向扑救,电脑随机方向射门。 第5轮结束之后,如果得分不同,比赛结束。 5轮之内,如果一方即使踢进剩下所有球,也无法达到另一方当前得分,比赛结束。 5论之后平分,比赛继续进行,直到某一轮分出胜负。
官方解答:我打算从今天开始,每天说一点这个小游戏的做法。方法有很多种,我只是提供一种参考。你可以按照自己喜欢的方式去做,那样她才是属于你的游戏。 先说一下方向的设定。我的想法比较简单,就是左、中、右三个方向,用字符串来表示。射门或者扑救的时候,直接输入方向。所以这里我准备用 input。有同学是用1-9的数字来表示八个方向和原地不动,每次输入一个数字,这也是可以的。不过这样守门员要扑住的概率可就小多了。 至于电脑随机挑选方向,如果你是用数字表示,就用我们之前讲过的randint来随机就行。不过我这次打算用random的另一个方法:choice。它的作用是从一个list中随机挑选一个元素。 于是,罚球的过程可以这样写:
from random import choice print ('Choose one side to shoot:') print ('left, center, right') you = input() print ('You kicked ' + you) direction = ['left', 'center', 'right'] com = choice(direction) print ('Computer saved ' + com) if you != com: print ('Goal!') else: print ('Oops...')
反之亦然,不赘述。
昨天有了一次罚球的过程,今天我就让它循环5次,并且记录下得分。先不判断胜负。用score_you表示你的得分,score_com表示电脑得分。开始都为0,每进一球就加1。
from random import choice score_you = 0 score_com = 0 direction = ['left', 'center', 'right'] for i in range(5): print ('==== Round %d - You Kick! ====' % (i+1)) print ('Choose one side to shoot:') print ('left, center, right') you = input() print ('You kicked ' + you) com = choice(direction) print ('Computer saved ' + com) if you != com: print ('Goal!') score_you += 1 else: print ('Oops...') print ('Score: %d(you) - %d(com)\n' % (score_you, score_com)) print ('==== Round %d - You Save! ====' % (i+1)) print ('Choose one side to save:') print ('left, center, right') you = input() print ('You saved ' + you) com = choice(direction) print ('Computer kicked ' + com) if you == com: print ('Saved!') else: print ('Oops...') score_com += 1 print ('Score: %d(you) - %d(com)\n' % (score_you, score_com))
在昨天代码的基础上,我们加上胜负判断,如果5轮结束之后是平分,就继续踢。 所以我们把一轮的过程单独拿出来作为一个函数kick,在5次循环之后再加上一个while循环。 另外,这里把之前的score_you和score_com合并成了一个score数组。这里的原因是,要让kick函数里用到外部定义的变量,需要使用全局变量的概念。暂时想避免说这个,而用list不存在这个问题。(但希望你不要觉得在函数里使用外面定义的变量很自然,后面我们会具体分析)
from random import choice score = [0, 0] direction = ['left', 'center', 'right'] def kick(): print('==== You Kick! ====') print('Choose one side to shoot:') print('left, center, right') you = input() print('You kicked ' + you) com = choice(direction) print('Computer saved ' + com) if you != com: print('Goal!') score[0] += 1 else: print('Oops...') print('Score: %d(you) - %d(com)\n' % (score[0], score[1])) print('==== You Save! ====') print('Choose one side to save:') print('left, center, right') you = input() print('You saved ' + you) com = choice(direction) print('Computer kicked ' + com) if you == com: print('Saved!') else: print('Oops...') score[1] += 1 print('Score: %d(you) - %d(com)\n' % (score[0], score[1])) for i in range(5): print('==== Round %d ====' % (i + 1)) kick() while (score[0] == score[1]): i += 1 print('==== Round %d ====' % (i + 1)) kick() if score[0] > score[1]: print('You Win!') else: print('You Lose.')
处理文件中的数据
比如我现在拿到一份文档,里面有某个班级里所有学生的平时作业成绩。因为每个人交作业的次数不一样,所以成绩的数目也不同,没交作业的时候就没有分。我现在需要统计每个学生的平时作业总得分。
文件 scores.txt
刘备 23 35 44 47 51
关羽 60 77 68
张飞 97 99 89 91
诸葛亮 100
f = open('scores.txt', encoding='gbk') lines = f.readlines() # print(lines) f.close() results = [] for line in lines: # print (line) data = line.split() # print (data) sum = 0 score_list = data[1:] for score in score_list: sum += int(score) result = '%s \t: %d\n' % (data[0], sum) # print (result) results.append(result) # print (results) output = open('result.txt', 'w', encoding='gbk') output.writelines(results) output.close()
查天气
import requests while True: city = input('请输入城市,回车退出:\n') if not city: break try: req = requests.get('http://wthrcdn.etouch.cn/weather_mini?city=%s' % city) except: print('查询失败') break #print(req.text) dic_city = req.json() #转换成字典 #print(dic_city) city_data = dic_city.get('data') #没有‘data’的话返回None if city_data: city_forecast = city_data['forecast'][0] #下面都可以换成get的方法 print(city_forecast.get('date')) print(city_forecast.get('high')) print(city_forecast.get('low')) print(city_forecast.get('type')) else: print('未获得')
注: city_data数据类型是字典
分数等级
输入一个分数。分数在 0-100 之间。90 以上是 A,80 以上是 B,70 以上是 C,60 以上是 D。60 以下是 E。
注://表示除法,求结果的整数(舍掉小数)。
循环嵌套的用法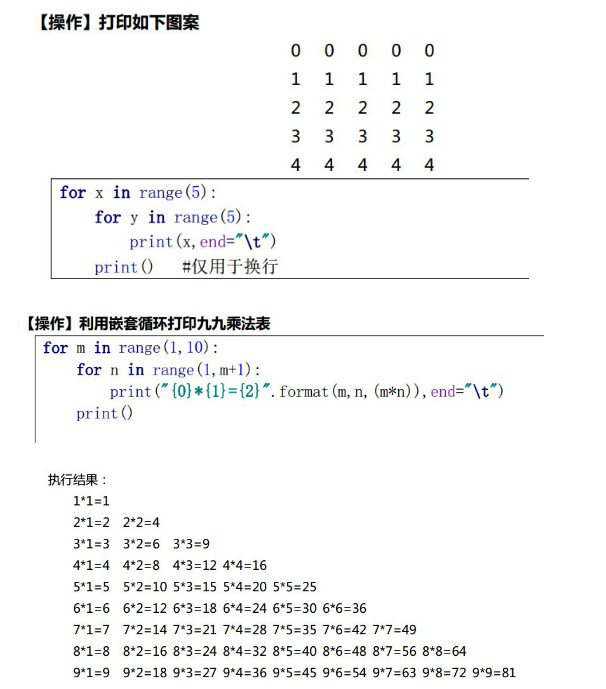
输入工资(注意else用法)
salarySum = 0 salarys =[] for i in range(4): s = input("请输入一共4名员工的薪资(按Q或q中途结束)") if s.upper() == "Q": print("录入完成,退出") break if float(s) < 0: continue salarys.append(float(s)) salarySum += float(s) else: # else语句,如果前面出现了Q/q(提前跳出循环),那么才不执行else print("您已经全部录入4名员工的薪资") print("录入平均薪资: ",salarys) print("平均薪资{0}".format(salarySum/4))